Here is script to add filter dropdown to a widget. We can filter to a particular item by selecting an item from the list.
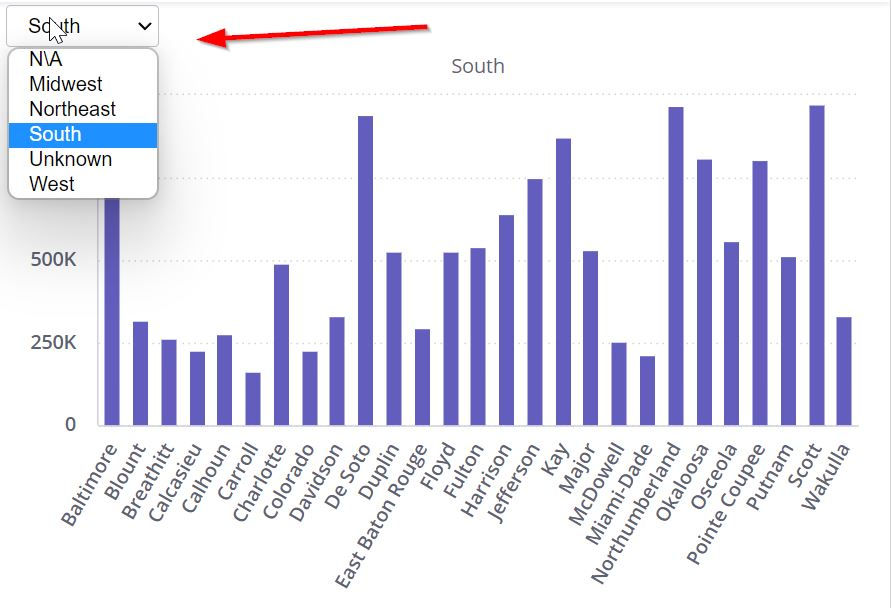
Steps:
Create a Line/Column/Bar/Area/Pie chart
Add below script to the widget and update the variable 'dimension' with name of dimension in the format "[Table Name.Column Name]". Items in this field will be displayed as button. Add the same column in widget filter pane and select one item in it.
Save the script and refresh widget.
widget.on('processresult', function(se, ev){
ev.result.chart.spacing = [30, 20, 77, 20 ]
});
var dimension = '[Records.Region]'
widget.on("domready", function(w){
var chart = w.chart[0][Object.keys(w.chart[0])[0]].hc
let items = getItemList(w).values;
let itemlist = Array.from(items, x=>x[0].data)
let widgetFilter = widget.metadata.panels[3].items.find(el => el.jaql.dim == dimension)
var chartContainer = $('.highcharts-container', element)
var dropdownHTML = `<select style="padding: 5px 10px; background:#FFFFFF; border: 1px solid #c0c1c2; margin:2px 5px;" name="select" id="select-${w.oid}"></select>`
chartContainer.before(dropdownHTML)
$.each(itemlist, function(index, value){
$(`#select-${w.oid}`).append(`<option value=${value}>${value}</option>`)
})
chartContainer.height(chartContainer.height() - 77);
var selecteValue = itemlist[0]
console.log(widgetFilter)
if(widgetFilter && widgetFilter.jaql.filter && widgetFilter.jaql.filter.members)
selecteValue = widgetFilter.jaql.filter.members[0]
document.getElementById(`select-${widget.oid}`).value = selecteValue
var select = document.getElementById(`select-${widget.oid}`);
select.addEventListener('change', (e) => {
console.log(e)
var selectedItem = e.target.value
widgetFilter.jaql.filter.members[0] = selectedItem
widget.refresh();
});
});
function runHTTP(jaql) {
// Use $internalHttp service if exists
const $internalHttp = prism.$injector.has("base.factories.internalHttp") ?
prism.$injector.get("base.factories.internalHttp") : null;
// Ajax configurations
const ajaxConfig = {
url: "/api/datasources/" + encodeURIComponent(jaql.datasource.title) + "/jaql",
method: "POST",
data: JSON.stringify(jaql),
contentType: "application/json",
dataType: "json",
async: false
};
// Use $internalHttp service for v8.0.1+
// else use default ajax request
const httpPromise = $internalHttp ? $internalHttp(ajaxConfig, true) : $.ajax(ajaxConfig);
// Return response
return httpPromise.responseJSON;
};
function getItemList(widget) {
const query = {
"datasource": widget.datasource.title,
"metadata": [
{
"jaql": {
"dim": dimension,
"filter": {
"explicit": false,
"multiSelection": true,
"all": true
}
}
}
]
};
return runHTTP(query);
}
Here's an updated version that adds an "All" option to the dropdown, and makes sure that it's the default selection if the dashboard is reloaded. This also incorporates the fix from my previous comment (about adding quotes so it can handle multi-word filter values). FYI - I used ChatGPT to help with modifying the code, and it's the one that inserted the various comments, so apologies if they're not accurate.
widget.on('processresult', function(se, ev){
ev.result.chart.spacing = [30, 20, 77, 20 ]
});
var dimension = '[Records.Region]'
widget.on("domready", function(w){
var chart = w.chart[0][Object.keys(w.chart[0])[0]].hc
let items = getItemList(w).values;
let itemlist = Array.from(items, x=>x[0].data)
let widgetFilter = widget.metadata.panels[3].items.find(el => el.jaql.dim == dimension)
var chartContainer = $('.highcharts-container', element)
// Create…
Hello! The script seems to work as expected, but it looks like it breaks if you choose a filter value that has any spaces in it. For instance, it works fine if I select things like "Litigation" or "eDiscovery", since those are just one word, but if I pick "Employee Relations", the widget goes blank and says No Results. If I click into the widget and look at the filter, it appears the script set it to just the word "Employee" by itself (which isn't a valid option, thus why it shows no results). How can we update the script to handle filter values that have spaces in them?