Here is an example of calling a rest API using Blox and displaying its response. This example is to display a list of Sisense folders owned by the logged in user. You can use any other url instead.
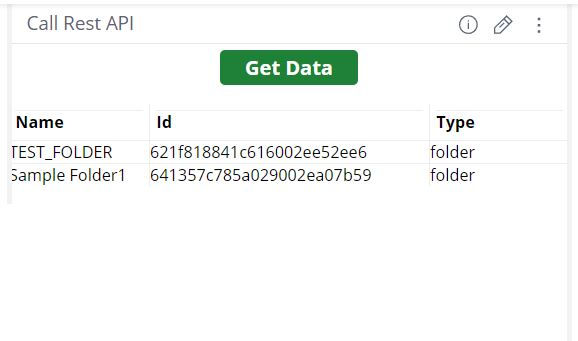
Steps:
Create a blox widget with 3 value panels, Name, Id and Type.
Type 0 instead of formula in all panels as in the screenshot below.
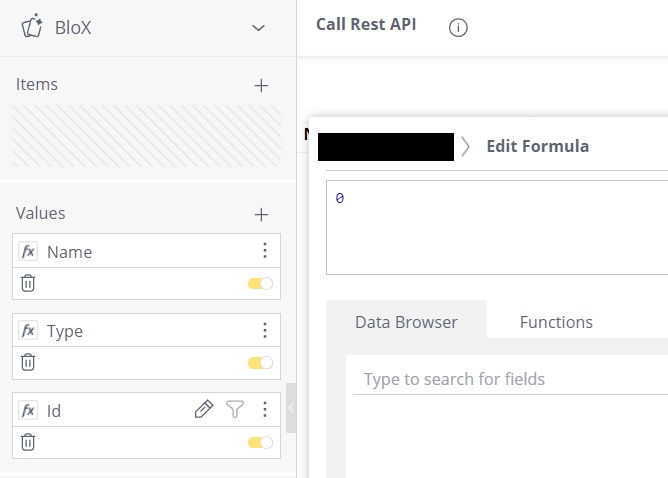
2. Copy and paste below blox script. In the below script, replace "/api/folders" (line 21) with any other URL you need to call.
Blox Script:
{
"style": ".blox-slides:not(:first-child) .fetch-button{display:none; !important;} .blox-slides .btn{margin-bottom:10px; font-weight:bold; color:black; font-size:1em}",
"script": "",
"title": "",
"carouselAnimation": {
"delay": 0
},
"body": [
{
"type": "ActionSet",
"class": "fetch-button",
"style": {
"padding": "5px"
},
"actions": [
{
"type": "fetchFromAPI",
"class": "fetch-button",
"title": "Get Data",
"data": {
"apiUrl": "/api/folders"
},
"style": {
"background-color": "#1f8038",
"color": "#ffffff"
}
}
]
},
{
"type": "ColumnSet",
"separator": false,
"columns": [
{
"type": "Column",
"width": "25%",
"separator": true,
"items": [
{
"type": "TextBlock",
"class": "fetch-button",
"weight": "light",
"horizontalAlignment": "left",
"separator": true,
"text": "Name",
"style": {
"font-size": "13px",
"font-weight": "bold",
"margin": "5px"
}
},
{
"type": "TextBlock",
"weight": "light",
"horizontalAlignment": "left",
"separator": true,
"text": "{panel:Name}",
"style": {
"font-size": "13px"
}
}
]
},
{
"type": "Column",
"separator": true,
"width": "50%",
"items": [
{
"type": "TextBlock",
"class": "fetch-button",
"weight": "light",
"horizontalAlignment": "left",
"separator": true,
"text": "Id",
"style": {
"font-size": "13px",
"font-weight": "bold",
"margin": "5px"
}
},
{
"type": "TextBlock",
"horizontalAlignment": "left",
"separator": true,
"weight": "light",
"text": "{panel:Type}",
"style": {
"font-size": "13px"
}
}
]
},
{
"type": "Column",
"separator": true,
"width": "25%",
"items": [
{
"type": "TextBlock",
"class": "fetch-button",
"weight": "light",
"horizontalAlignment": "left",
"separator": true,
"text": "Type",
"style": {
"font-size": "13px",
"font-weight": "bold",
"margin": "5px"
}
},
{
"type": "TextBlock",
"weight": "light",
"horizontalAlignment": "left",
"separator": true,
"color": "default",
"text": "{panel:Id}",
"style": {
"font-size": "13px"
}
}
]
}
]
}
]
}
Configuration:
{
"fontFamily": "Open Sans",
"fontSizes": {
"default": 16,
"small": 12,
"medium": 22,
"large": 32,
"extraLarge": 50
},
"fontWeights": {
"default": 500,
"light": 100,
"bold": 1000
},
"containerStyles": {
"default": {
"backgroundColor": "#ffffff",
"foregroundColors": {
"default": {
"normal": "#000000"
},
"white": {
"normal": "#000000"
},
"grey": {
"normal": "#3A4356"
},
"orange": {
"normal": "#f2B900"
},
"yellow": {
"normal": "#ffcb05"
},
"black": {
"normal": "#000000"
},
"lightGreen": {
"normal": "#3ADCCA"
},
"green": {
"normal": "#54a254"
},
"red": {
"normal": "#dd1111"
},
"accent": {
"normal": "#2E89FC"
},
"good": {
"normal": "#54a254"
},
"warning": {
"normal": "#e69500"
},
"attention": {
"normal": "#cc3300"
}
}
}
},
"imageSizes": {
"default": 40,
"small": 40,
"medium": 80,
"large": 160
},
"imageSet": {
"imageSize": "medium",
"maxImageHeight": 100
},
"actions": {
"color": "",
"backgroundColor": "white",
"maxActions": 5,
"spacing": "extraLarge",
"buttonSpacing": 5,
"actionsOrientation": "horizontal",
"actionAlignment": "center",
"showCard": {
"actionMode": "inline",
"inlineTopMargin": 16,
"style": "default"
}
},
"spacing": {
"default": 5,
"small": 20,
"medium": 60,
"large": 20,
"extraLarge": 40,
"padding": 2
},
"separator": {
"lineThickness": 1,
"lineColor": "#eeeeee"
},
"factSet": {
"title": {
"size": "default",
"color": "default",
"weight": "bold",
"warp": true
},
"value": {
"size": "default",
"color": "default",
"weight": "default",
"warp": true
},
"spacing": 20
},
"supportsInteractivity": true,
"imageBaseUrl": "",
"height": 618
}
3. Create a custom action with name 'fetchFromAPI' and use below script. Here we are pushing the response from API to widget. So panel names specified in script should be same as in Blox widget.
const apiUrl = payload.data.apiUrl;
const $internalHttp = prism.$injector.has("base.factories.internalHttp") ?
prism.$injector.get("base.factories.internalHttp") : null;
// Ajax configurations
const ajaxConfig = {
url: apiUrl,
method: "GET",
contentType: "application/json",
accept: "application/json",
async: false
};
const httpPromise = $internalHttp ? $internalHttp(ajaxConfig, true) : $.ajax(ajaxConfig);
payload.widget.on('processresult', function(se, ev){
ev.result.length = 0
$.each(httpPromise.responseJSON, function(index, value){
ev.result.push({
"0": {
"Panel": "Name",
"Value": value.name,
"Text": value.name,
"HasValue": true
},
"1": {
"Panel": "Id",
"Value": value.type,
"Text": value.type,
"HasValue": true
},
"2": {
"Panel": "Type",
"Value": value.oid,
"Text": value.oid,
"HasValue": true
}
})
})
})
payload.widget.refresh()
4. Add below widget script. This is to hide '0' (which we specified in value panel formula)
widget.on('processresult', function(se, ev){
$.each(ev.result[0], function(index, value){
value.Value = ''
value.Text = ''
})
})
留言